Python is a popular programming language among beginners and seasoned programmers due to its well-known simplicity and ease of use. Even with its simple syntax, many developers, especially those unfamiliar with the language, commonly make mistakes that can negatively affect their code’s functionality, readability, and performance. Whether you’ve been using Python for years or are just getting started, knowing these pitfalls to avoid will help you become a better programmer and make more effective code. A Programming Course can further enhance your skills by teaching you best practices and avoiding common mistakes in Python programming.
This blog will examine five common Python Programming mistakes and how to fix them.
Table of Contents
- Not Using List Comprehensions
- Misusing Mutable Default Arguments
- Not Handling Exceptions Properly
- Using Global Variables Unnecessarily
- Ignoring Python Standard Library
- Conclusion
Not Using List Comprehensions
Not utilising list comprehensions is one of the most frequent errors made by Python developers. To produce condensed lists, list comprehensions apply an expression to each element in an iterable, such as a list or range. This feature makes your code more efficient and easier to read.
Many developers—especially those who come from other programming languages—employ loops to create or change lists, which can produce lengthy and intricate code.
An example of a standard loop that would produce a list of squares would be as follows:

List comprehensions are an essential Python feature because they speed up computation and minimise the amount of code needed.
Although this works, a list comprehension can do the same goal more gracefully:

List comprehensions are an essential Python feature because they speed up computation and minimise the amount of code needed.
How to Avoid This Mistake
Learn about list comprehensions and apply them when building lists from preexisting sequences. They’ll help to improve the readability and clarity of your code.
Misusing Mutable Default Arguments
One of Python tougher features is its handling of default arguments, especially modified default arguments. Unexpected behaviour might arise when changeable objects, such as dictionaries or lists, are used as default parameters. When a function is defined, it is evaluated only once; it is not evaluated each time it is called. This implies that any changes you make to the mutable object will be reflected in all ensuing calls to the function.
Here’s an example of how this mistake might manifest:
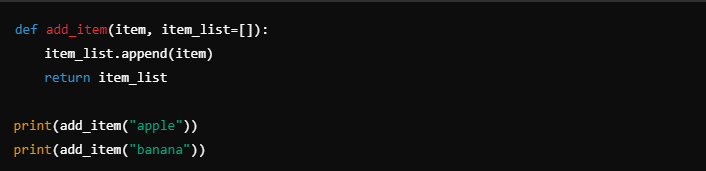
You might expect add_item to return a new list each time, but the result will be:

Each call modifies the default list; thus, the changes continue between calls and may result in perplexing issues.
How to Avoid This Mistake
Initialise the mutable object inside the method after using None as the default argument:
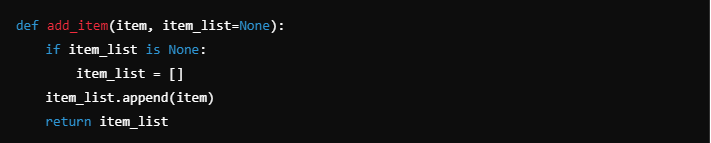
Now, a new list is generated each time the function is run.
Not Handling Exceptions Properly
Though many developers don’t use it effectively, Python try, except, and finally, blocks for exception handling are immensely powerful. Some people make the error of catching exceptions too broadly, which can make it challenging to figure out where the code went wrong. Some completely neglect to handle exceptions, which might result in an unexpected program crash.
One typical mistake is to capture all exceptions in the manner shown below:

This method captures all anomalies, making it challenging to identify the precise problem. Additionally, it stops the application from offering helpful details about what went wrong.
How to Avoid This Mistake
It is best to address exceptions that are special to you and allow others to arise naturally. As an illustration:
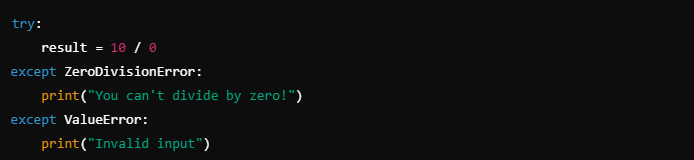
In this manner, you catch only the exceptions that you are familiar with handling and let other parts of the software handle others.
Using Global Variables Unnecessarily
In Python, using global variables is typically seen as bad practice since it can make your code more difficult to read and maintain. Because global variables are accessible and adaptable from anywhere in the code, errors and unexpected side effects may result.
Here’s an example of using a global variable:
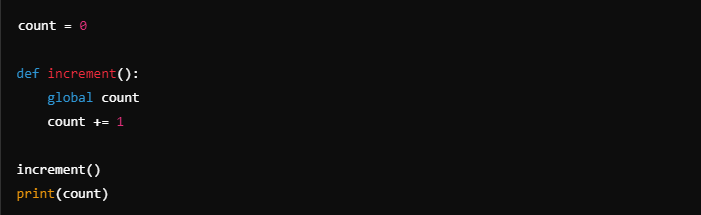
Although this can appear practical, it produces difficult-to-manage code, particularly in larger systems. Because any function can alter global variables, often in complex ways to track down, they can easily result in unanticipated behaviour.
How to Avoid This Mistake
Global variables should be avoided wherever possible. Alternatively, if data must be shared between methods, utilise class properties or send values to functions explicitly:
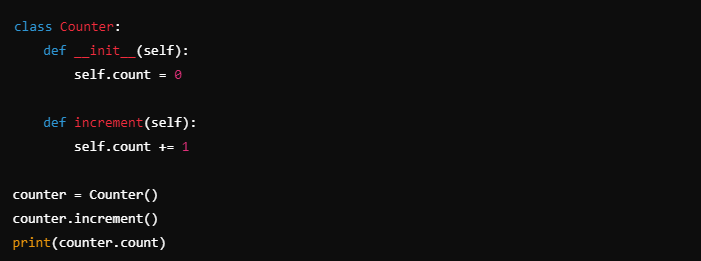
By encapsulating the state, this technique simplifies and manages your code.
Ignoring Python Standard Library
One of Python most significant assets is its standard library, which offers a multitude of modules and functions that can save time and effort. However, many developers, particularly novices, either ignore or don’t know about the standard library’s resources. They consequently write new code to do tasks that Python already handles. For example, you can utilise the zipfile module rather than creating your code to handle file compression:

The standard Python library includes functions for handling regular expressions (re), modifying file paths (os and shutil), and working with dates (DateTime).
How to Avoid This Mistake
Look through Python standard library before creating your solution to a problem. It’s likely that a module that fulfils your requirements already exists.
Conclusion
Although Python is a powerful and versatile language, even experienced programmers make common mistakes. You can write cleaner, more efficient, and maintainable code by recognising the above-mentioned critical errors.
Mastering Python best practices ensures your code remains elegant, efficient, and functional. Avoiding these mistakes will help you achieve Python proficiency, no matter your experience level. The Knowledge Academy offers free resources that dive deep into Python programming best practices and techniques for those looking to enhance their skills further.